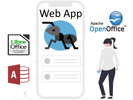
The GARDENA Smart System, developed by Husqvarna Group, provides innovative solutions for managing and controlling your garden and outdoor devices. To integrate and interact with the GARDENA Smart System, developers can leverage the Husqvarna Group's Open API, which offers a convenient way to access and control various smart devices using RESTful web services and JSON data format.
In this guide, we will show ypou how to connect to the GARDENA smart system using ASP.NET, a popular framework for building web applications. By utilizing the power of RESTful APIs and JSON, we can seamlessly communicate with GARDENA devices, retrieve information, and perform actions remotely.
What to do first:
Obtaining API credentials (client ID and client secret) at
https://developer.husqvarnagroup.cloud/
Implementing the authentication flow to obtain an access token.
Now you can:
Retrieving a list of available devices and their properties.
Controlling device states, such as turning on/off or adjusting settings.
Monitoring device status and retrieving real-time data.
Handling JSON Data:
Parsing JSON responses from the GARDENA API.
Serializing JSON data to send requests and update device settings.
Posible Common Use Cases:
Creating schedules for automated device operations.
Managing device groups and zones for efficient control.
Handling events and notifications from the GARDENA system.
Note: Before starting the implementation, make sure to register as a developer and obtain API credentials from the Husqvarna Group's developer portal. Familiarity with ASP.NET, RESTful APIs, and JSON will be helpful throughout the development process.
Private Sub doAuth2()
Dim clientId As String = "xxxxxx-xxx-xxxx-xxxx-xx"
Dim clientSecret As String = "xxxxx-xxxx-xxxx-xxxx-xxxxxxxxxx"
Dim accessToken As String = GetAccessToken(clientId, clientSecret)
Dim LocationID As String = "xxxxxxx-xxxx-xxxx-xxx-xxxxxxxx"
Response.Write(accessToken)
Response.Write(GetLanMoverList(accessToken, clientId))
Response.Write(GetLocations(accessToken, LocationID, clientId))
'End If
End Sub
Private Function GetAccessToken(clientId As String, clientSecret As String) As String
Dim tokenEndpoint As String = "https://api.authentication.husqvarnagroup.dev/v1/oauth2/token"
Dim redirectUri As String = "https://localhost:44306/"
Dim request As HttpWebRequest = CType(WebRequest.Create(tokenEndpoint), HttpWebRequest)
request.Method = "POST"
Dim postData As String = String.Format("grant_type=client_credentials&client_id={0}&client_secret={1}&redirect_uri={2}", clientId, clientSecret, redirectUri)
Dim byteArray As Byte() = Encoding.UTF8.GetBytes(postData)
request.ContentType = "application/x-www-form-urlencoded"
request.ContentLength = byteArray.Length
Dim dataStream As Stream = request.GetRequestStream()
dataStream.Write(byteArray, 0, byteArray.Length)
dataStream.Close()
Dim response As WebResponse = request.GetResponse()
dataStream = response.GetResponseStream()
Dim reader As New StreamReader(dataStream)
Dim responseFromServer As String = reader.ReadToEnd()
Dim serializer As New JavaScriptSerializer()
Dim tokenData As Dictionary(Of String, Object) = serializer.Deserialize(Of Dictionary(Of String, Object))(responseFromServer)
If tokenData.ContainsKey("access_token") Then
Return tokenData("access_token").ToString()
Else
Return ""
End If
End Function
Private Function GetLanMoverList(Token As String, clientId As String) As String
Dim tokenEndpoint As String = "https://api.amc.husqvarna.dev/v1/mowers"
Dim X_Api_Key As String = clientId
Dim Token_var As String = "Bearer " + Token
Dim Authorization_Provider As String = "husqvarna"
Dim request As HttpWebRequest = CType(WebRequest.Create(tokenEndpoint), HttpWebRequest)
request.Method = "GET"
request.Headers.Add("Authorization", Token_var)
request.Headers.Add("X-Api-Key", X_Api_Key)
request.Headers.Add("Authorization-Provider", Authorization_Provider)
Dim response As WebResponse = request.GetResponse()
Dim dataStream As Stream = response.GetResponseStream()
Dim reader As New StreamReader(dataStream)
Dim responseFromServer As String = reader.ReadToEnd()
reader.Close()
dataStream.Close()
response.Close()
Return responseFromServer
End Function
Private Function GetLocations(Token As String, LocationID As String, clientid As String) As String
Dim tokenEndpoint As String = "https://api.smart.gardena.dev/v1/locations" + "/" + LocationID
Dim X_Api_Key As String = clientid
Dim Token_var As String = "Bearer " + Token
Dim Authorization_Provider As String = "husqvarna"
Dim request As HttpWebRequest = CType(WebRequest.Create(tokenEndpoint), HttpWebRequest)
request.Method = "GET"
request.Headers.Add("Authorization", Token_var)
request.Headers.Add("X-Api-Key", X_Api_Key)
request.Headers.Add("Authorization-Provider", Authorization_Provider)
Dim response As WebResponse = request.GetResponse()
Dim dataStream As Stream = response.GetResponseStream()
Dim reader As New StreamReader(dataStream)
Dim responseFromServer As String = reader.ReadToEnd()
reader.Close()
dataStream.Close()
response.Close()
' Parse the JSON string into a JsonDocument
Dim jsonDocumentVar As JsonDocument = JsonDocument.Parse(responseFromServer)
Dim locationIdVar As String = jsonDocumentVar.RootElement.GetProperty("data").GetProperty("id").GetString()
Dim locationName As String = jsonDocumentVar.RootElement.GetProperty("data").GetProperty("attributes").GetProperty("name").GetString()
Return responseFromServer
End Function